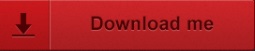
To share your experience and more insights on this topic, please leave your thoughts in the comments section below. If you need to perform processing on multiple items of the list in a single iteration then the overhead of iterations can be lesser in LinkedList than that of an ArrayList which involves copying array element multiple times. Traversing a linked list or inserting an item in the middle is very expensive as you have to iterate over each item and is very likely to have cache misses.
#FIXED SIZE ARRAY VS ARRAYLIST CODE#
The time to do random access is proportional to the size of the list (unless this is the first or the last element where the cost is fixed) - average case time complexity is O(n).Ĭonsider the following code examples for traversing a LinkedList The code below would be very slow as LinkedList does not support random access and there is a huge overhead while traversing for each iteration.Ī better approach for improved performance would be to use the following code instead.Īn ArrayList is faster and better as it supports random access to its elements. Random access has a fixed time - time complexity is O(1). It has a memory space overhead for storing the references to the previous and next element in the list for every element. It has a memory space overhead as the list of objects has to be preallocated which means empty elements at the end of the list. This just involves allocating an internal object, and the cost is uniform. Typically, this involves setting an internal array location to the element reference with time complexity of O(1)., but occasionally for the case of an overflow, it results in the array being reallocated and has a fixed averaged cost again involving triggering a call to System.arraycopy(), with worst-case time complexity of O(n).
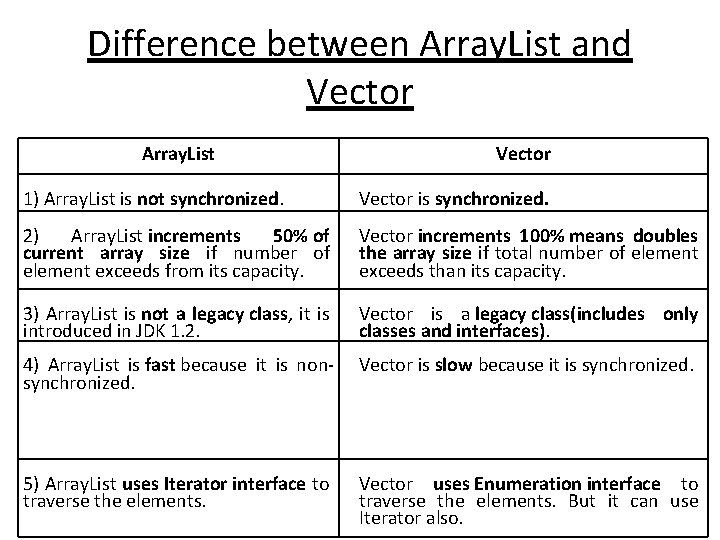
Adding (or removing) an element at the middle of the list would have a time complexity of O(n) as it would involve iterating over the list. This involves allocating (or deallocating) an internal record for the element and then realigning a couple of links and has a fixed cost.
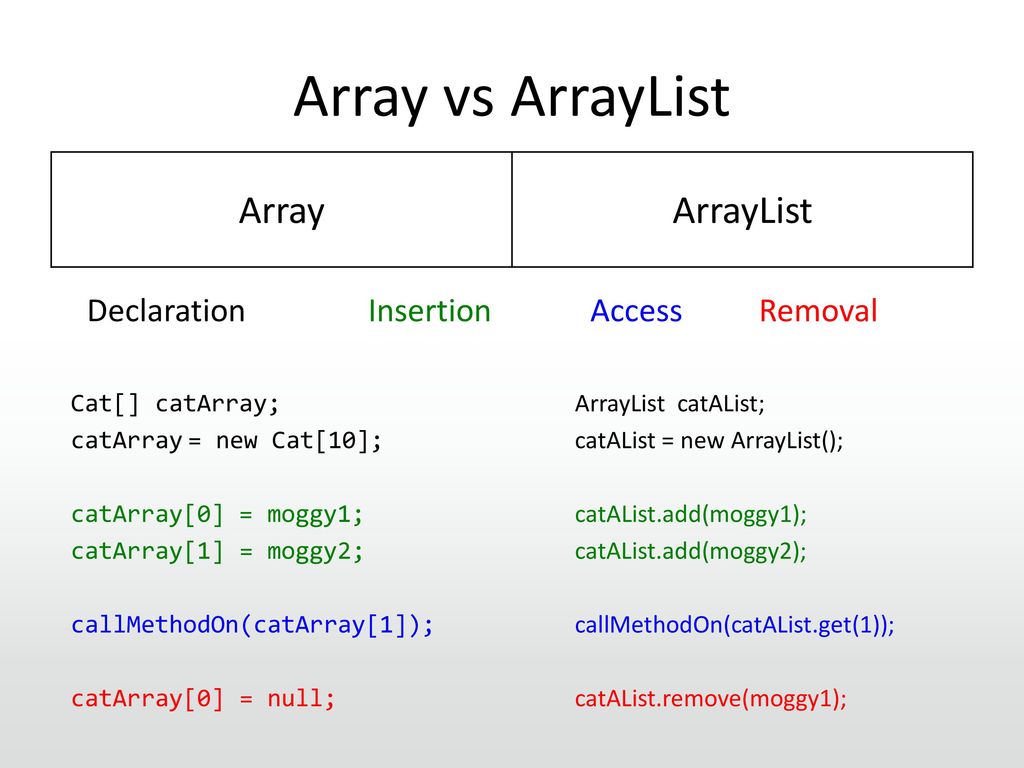
best case time complexity is O(1).Īverage-case time complexity O(n) as System.arraycopy() would be linear time. This involves moving all the existing elements back (or forward) by one place requiring copying of items done via a call to System.arraycopy(). Space and Time Complexity of Various Operations Characteristic LinkedList does not implement the RandomAccess interface, whereas implements the interface (instead of Deque interface). calling constructure ArrayList(initialCapacity)īy default, an creates a list of initial capacity 10, while LinkedList only constructs an empty list without any initial capacity. It also includes a method void trimToSize()to reduce the size based on existing elements. It is possible to specify the initial capacity of an ArrayList through the constructor ArrayList(int initialCapacity) and later increases the capacity using void ensureCapacity(int minCapacity), if necessary, to ensure that it can hold at least the number of elements specified by the minimum capacity argument. It is internally implemented as an object array, which can increase the size as necessary to support more number of elements in the collection. ArrayListĪrrayList is a resizable-array implementation of the List interface. Other interfaces it implements are Serializable, Cloneable, and Deque (with super-interface as Queue). The elements/ items in the data structure can be modified to change the size of the object as and when required. The size of the Array cannot be changed once the object has been defined. In a doubly-linked list, every node points to its previous and next node. Array ArrayList Size of data structure: An array contains a data structure of fixed length.
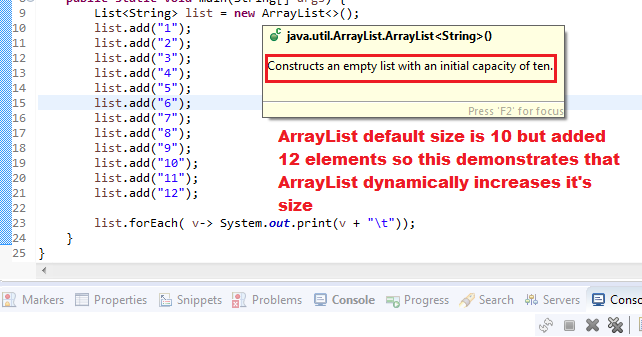
LinkedList in Java is a doubly-linked list implementation of the List interface. The linked list itself contains a reference to the first element of the list, which is called the head element. The last element (or tail) of the sequence points to a null element. The LinkedList data structure contains an ordered set of data elements (know as nodes) such that each element contains a link or reference to its successor ( next element). These span from calling helper methods to streaming the array and collecting the elements.Vector is similar to ArrayList except they are provided with automatic synchronization, which makes them thread-safe but causes some performance overhead. There are numerous ways to convert an array to an ArrayList in Java.

However, as Java 7 removed the need to explicitly set the type in the diamond operator, this became obsolete. This was really useful when you'd have a > list. Now, the key takeaway of this approach was that you don't need to specify the type when initializing an ArrayList. The asList() method returns the contents of the array in a List: Employee emp1 = new Employee( "John") Įmployee array = new Employee The Arrays helper class has a lot of useful methods. Let's start off with the simplest form of conversion.
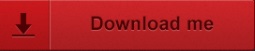